A library that provides access to Google Maps on Android and Apple Maps on iOS.
This library is currently in alpha and will frequently experience breaking changes. It is not available in the Expo Go app – use development builds to try it out.
Installation
-
npx expo install expo-maps
If you are installing this in an existing React Native app, make sure to install expo
in your project.
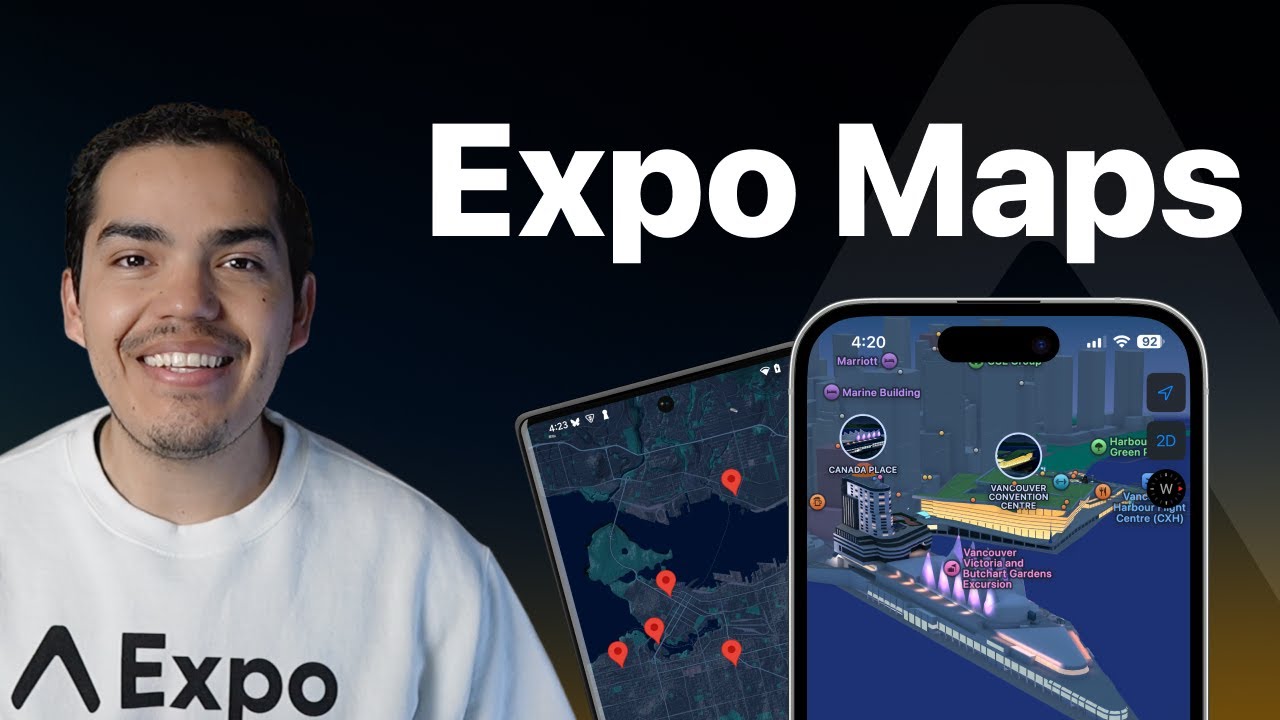
Configuration
Expo Maps provides access to the platform native map APIs on Android and iOS.
- Apple Maps (available on iOSonly). No additional configuration is required to use it after installing this package.
- Google Maps (available on Androidonly). While Google provides a Google Maps SDK for iOS, Expo Maps supports it exclusively on Android. If you want to use Google Maps on iOS, you can look into using an alternative library or writing your own.
Google Cloud API setup
Before you can use Google Maps on Android, you need to register a Google Cloud API project, enable the Maps SDK for Android, and add the associated configuration to your Expo project.
Set up Google Maps on Android
If you have already registered a project for another Google service on Android, such as Google Sign In, you enable the Maps SDK for Android on your project and jump to step 4.
1
Register a Google Cloud API project and enable the Maps SDK for Android
- Open your browser to the Google API Manager and create a project.
- Once it's created, go to the project and enable the Maps SDK for Android.
2
Copy your app's SHA-1 certificate fingerprint
- If you are deploying your app to the Google Play Store, you'll need to upload your app binary to Google Play console at least once. This is required for Google to generate your app signing credentials.
- Go to the Google Play Console > (your app) > Release > Setup > App integrity > App Signing.
- Copy the value of SHA-1 certificate fingerprint.
- If you have already created a development build, your project will be signed using a debug keystore.
- After the build is complete, go to your project's dashboard, then, under Configure > click Credentials.
- Under Application Identifiers, click your project's package name and under Android Keystore copy the value of SHA-1 Certificate Fingerprint.
3
Create an API key
- Go to Google Cloud Credential manager and click Create Credentials, then API Key.
- In the modal, click Edit API key.
- Under Key restrictions > Application restrictions, choose Android apps.
- Under Restrict usage to your Android apps, click Add an item.
- Add your
android.package
from app.json (for example:com.company.myapp
) to the package name field. - Then, add the SHA-1 certificate fingerprint's value from step 2.
- Click Done and then click Save.
4
Add the API key to your project
- Copy your API Key into your app.json under the
android.config.googleMaps.apiKey
field. - Create a new development build, and you can now use the Google Maps API on Android with
expo-maps
.
Permissions
To display the user's location on the map, you need to declare and request location permission beforehand. You can configure this using the built-in config plugin if you use config plugins in your project (EAS Build or npx expo run:[android|ios]
). The plugin allows you to configure various properties that cannot be set at runtime and require building a new app binary to take effect.
Example app.json with config plugin
{
"expo": {
"plugins": [
[
"expo-maps",
{
"requestLocationPermission": true,
"locationPermission": "Allow $(PRODUCT_NAME) to use your location"
}
]
]
}
}
Configurable properties
Name | Default | Description |
---|---|---|
requestLocationPermission | false | A boolean to add permissions to AndroidManifest.xml and Info.plist. |
locationPermission | "Allow $(PRODUCT_NAME) to use your location" | Only for: iOS A string to set the |
Usage
import { AppleMaps, GoogleMaps } from 'expo-maps';
import { Platform, Text } from 'react-native';
export default function App() {
if (Platform.OS === 'ios') {
return <AppleMaps.View style={{ flex: 1 }} />;
} else if (Platform.OS === 'android') {
return <GoogleMaps.View style={{ flex: 1 }} />;
} else {
return <Text>Maps are only available on Android and iOS</Text>;
}
}
API
import { AppleMaps, GoogleMaps } from 'expo-maps';
// ApplesMaps.View and GoogleMaps.View are the React components
Components
Type: React.Element<AppleMapsViewProps>
(event: {
bearing: number,
coordinates: Coordinates,
tilt: number,
zoom: number
}) => void
Lambda invoked when the map was moved by the user.
(event: {
coordinates: Coordinates
}) => void
Lambda invoked when the user clicks on the map. It won't be invoked if the user clicks on POI or a marker.
(event: AppleMapsMarker) => void
Lambda invoked when the marker is clicked
StyleProp<ViewStyle>
AppleMapsUISettings
The MapUiSettings
to be used for UI-specific settings on the map.
Type: React.Element<Omit<GoogleMapsViewProps, 'ref'>>
(event: {
bearing: number,
coordinates: Coordinates,
tilt: number,
zoom: number
}) => void
Lambda invoked when the map was moved by the user.
(event: {
coordinates: Coordinates
}) => void
Lambda invoked when the user clicks on the map. It won't be invoked if the user clicks on POI or a marker.
(event: {
coordinates: Coordinates
}) => void
Lambda invoked when the user long presses on the map.
(event: GoogleMapsMarker) => void
Lambda invoked when the marker is clicked
(event: {
coordinates: Coordinates,
name: string
}) => void
Lambda invoked when a POI is clicked.
Ref<GoogleMapsViewType>
StyleProp<ViewStyle>
GoogleMapsUISettings
The MapUiSettings
to be used for UI-specific settings on the map.
GoogleMapsUserLocation
User location, overrides default behavior.
Type: React.Element<GoogleStreetViewProps>
Coordinates
StyleProp<ViewStyle>
Hooks
Parameter | Type |
---|---|
options(optional) | PermissionHookOptions<object> |
Check or request permissions to access the location.
This uses both requestPermissionsAsync
and getPermissionsAsync
to interact with the permissions.
[null | PermissionResponse, RequestPermissionMethod<PermissionResponse>, GetPermissionMethod<PermissionResponse>]
Example
const [status, requestPermission] = useLocationPermissions();
Methods
Checks user's permissions for accessing location.
Promise<PermissionResponse>
A promise that fulfills with an object of type PermissionResponse
.
Asks the user to grant permissions for location.
Promise<PermissionResponse>
A promise that fulfills with an object of type PermissionResponse
.
Types
Type: AppleMapsMarker
extended by:
Property | Type | Description |
---|---|---|
backgroundColor(optional) | string | The background color of the annotation. |
icon(optional) | SharedRefType<'image'> | The custom icon to display in the annotation. |
text(optional) | string | The text to display in the annotation. |
textColor(optional) | string | The text color of the annotation. |
Property | Type | Description |
---|---|---|
coordinates(optional) | Coordinates | The coordinates of the marker. |
systemImage(optional) | string | The SF Symbol to display for the marker. |
tintColor(optional) | string | The tint color of the marker. |
title(optional) | string | The title of the marker, displayed in the callout when the marker is clicked. |
Property | Type | Description |
---|---|---|
isTrafficEnabled(optional) | boolean | Whether the traffic layer is enabled on the map. |
mapType(optional) | AppleMapsMapType | Defines which map type should be used. |
selectionEnabled(optional) | boolean | If true, the user can select a location on the map to get more information. |
Property | Type | Description |
---|---|---|
compassEnabled(optional) | boolean | Whether the compass is enabled on the map. If enabled, the compass is only visible when the map is rotated. |
myLocationButtonEnabled(optional) | boolean | Whether the my location button is visible. |
scaleBarEnabled(optional) | boolean | Whether the scale bar is displayed when zooming. |
togglePitchEnabled(optional) | boolean | Whether the user is allowed to change the pitch type. |
Property | Type | Description |
---|---|---|
coordinates(optional) | Coordinates | The middle point of the camera. |
zoom(optional) | number | The zoom level of the camera. For some view sizes, lower zoom levels might not be available. |
Property | Type | Description |
---|---|---|
latitude(optional) | number | The latitude of the coordinate. |
longitude(optional) | number | The longitude of the coordinate. |
Property | Type | Description |
---|---|---|
coordinates(optional) | Coordinates | The coordinates of the marker. |
draggable(optional) | boolean | Whether the marker is draggable. |
icon(optional) | SharedRefType<'image'> | The custom icon to display for the marker. |
showCallout(optional) | boolean | Whether the callout should be shown when the marker is clicked. |
snippet(optional) | string | The snippet of the marker, Displayed in the callout when the marker is clicked. |
title(optional) | string | The title of the marker, displayed in the callout when the marker is clicked. |
Property | Type | Description |
---|---|---|
isBuildingEnabled(optional) | boolean | Whether the building layer is enabled on the map. |
isIndoorEnabled(optional) | boolean | Whether the indoor layer is enabled on the map. |
isMyLocationEnabled(optional) | boolean | Whether finding the user's location is enabled on the map. |
isTrafficEnabled(optional) | boolean | Whether the traffic layer is enabled on the map. |
mapType(optional) | GoogleMapsMapType | Defines which map type should be used. |
maxZoomPreference(optional) | number | The maximum zoom level for the map. |
minZoomPreference(optional) | number | The minimum zoom level for the map. |
selectionEnabled(optional) | boolean | If true, the user can select a location on the map to get more information. |
Property | Type | Description |
---|---|---|
compassEnabled(optional) | boolean | Whether the compass is enabled on the map. If enabled, the compass is only visible when the map is rotated. |
indoorLevelPickerEnabled(optional) | boolean | Whether the indoor level picker is enabled . |
mapToolbarEnabled(optional) | boolean | Whether the map toolbar is visible. |
myLocationButtonEnabled(optional) | boolean | Whether the my location button is visible. |
rotationGesturesEnabled(optional) | boolean | Whether rotate gestures are enabled. |
scaleBarEnabled(optional) | boolean | Whether the scale bar is displayed when zooming. |
scrollGesturesEnabled(optional) | boolean | Whether the scroll gestures are enabled. |
scrollGesturesEnabledDuringRotateOrZoom(optional) | boolean | Whether the scroll gestures are enabled during rotation or zoom. |
tiltGesturesEnabled(optional) | boolean | Whether the tilt gestures are enabled. |
togglePitchEnabled(optional) | boolean | Whether the user is allowed to change the pitch type. |
zoomControlsEnabled(optional) | boolean | Whether the zoom controls are visible. |
zoomGesturesEnabled(optional) | boolean | Whether the zoom gestures are enabled. |
Property | Type | Description |
---|---|---|
coordinates | Coordinates | User location coordinates. |
followUserLocation | boolean | Should the camera follow the users' location. |
Property | Type | Description |
---|---|---|
setCameraPosition | (config: SetCameraPositionConfig) => void | Update camera position. config: SetCameraPositionConfig New camera position config. |
Type: CameraPosition
extended by:
Property | Type | Description |
---|---|---|
duration(optional) | number | The duration of the animation in milliseconds. |
Enums
The type of map to display.
AppleMapsMapType.HYBRID = "HYBRID"
A satellite image of the area with road and road name layers on top.
The type of map to display.
GoogleMapsMapType.HYBRID = "HYBRID"
Satellite imagery with roads and points of interest overlayed.
Permissions
Android
To show the user's location on the map, the expo-maps
library requires the following permissions:
ACCESS_COARSE_LOCATION
: for approximate device locationACCESS_FINE_LOCATION
: for precise device location
Android Permission | Description |
---|---|
Allows an app to access approximate location.
| |
Allows an app to access precise location.
| |
Allows a regular application to use Service.startForeground.
| |
Allows a regular application to use Service.startForeground with the type "location".
| |
Allows an app to access location in the background.
|
iOS
The following usage description keys are used by this library: